In this article, we explain what is Maven and how Maven projects are structured. We recommend to use Maven projects to create new plugins for Icy.
Maven is a project management tool. Here’s what we can read from the official What is Maven Apache web site :
<<Its primary goal is to allow a developer to comprehend the complete state of a development effort in the shortest period of time. In order to attain this goal, Maven deals with several areas of concern:
- Making the build process easy
- Providing a uniform build system
- Providing quality project information
- Encouraging better development practices>>
That said and to be truly honest, Maven is a big beast which can do a lot of stuff for you but it takes ages to master it. Fortunately, you don’t need to master fully Maven in order to use it for your Icy plugin developments. We will focus here on explaining the basics so you can get quickly started.
Bu before starting, here’s two alternative quick Maven introductions that may interest you eventually:
ImageJ Maven introduction
Maven in 5 minutes (Apache)
Maven project structure
Maven uses the notion of Convention over Configuration. In other words, to use Maven in your projects, you must adopt its architecture. The architecture of a Maven project consists of a project directory with a POM file (pom.xml) containing all project information at the root and one /src folder containing the source code (in /main) and the test files (in /test). The POM file is used by Maven program (mvn) to build your project. We detail below its content.
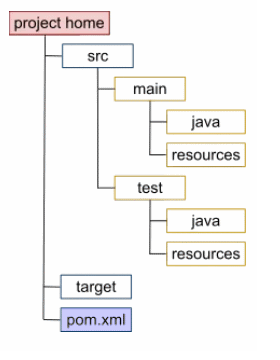
- project_home/src/main/java
main java source files of the project - project_home/src/main/resources
main resources files (icons, images, xml..) - project_home/src/test/java
java source files used for test only - project_home/src/test/resources
resources files used for test only - project_home/target
build destination (contains generated .class / .jar files) - project_home/pom.xml
POM configuration file of the project
POM file description
The POM file is an XML file that contains all project information and instructions for the Maven program (mvn) to build the project. Here is a quick description of the POM file with the main sections and how to fill them:
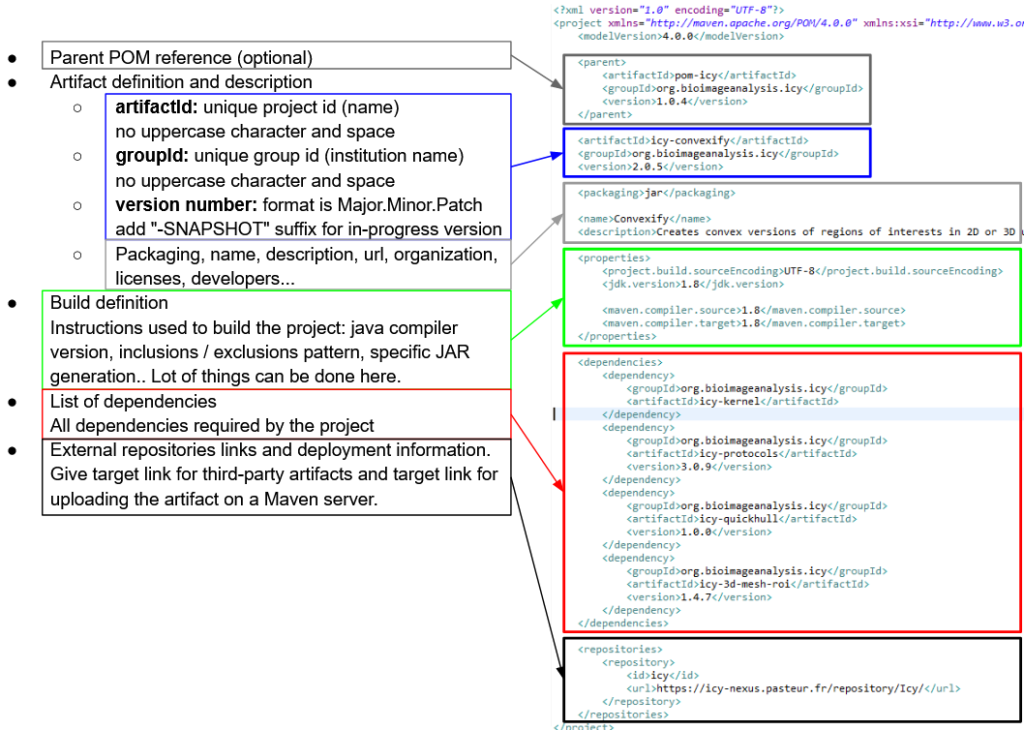
Again, here we provide just the basics to get a quick overview of what is Maven. We provide more information in dedicated articles as create a new plugin for Icy. If you are really interested in getting more details about the POM file you can find a more complete introduction to POM file as well as a full reference on the Apache Maven website.
To give you a better idea of what can contain a POM file, we inserted three examples below. We also invite you to continue your reading with this article on how to create a new plugin for Icy and this article on how to migrate an old Icy plugin to Maven.
- POM template for Icy Plugins
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <!-- Inherited Icy Parent POM --> <parent> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>parent-pom-plugin</artifactId> <version>1.0.1</version> </parent> <!-- Project Information --> <artifactId>IcyPluginTemplate</artifactId> <version>1.0.0-SNAPSHOT</version> <packaging>jar</packaging> <name>A template for Icy plugins.</name> <description>This repo serves as a template for you to implement new Icy plugins.</description> <url></url> <inceptionYear>2020</inceptionYear> <organization> <name>MyOrganizationName</name> <url>https://myorganizationwebsite.net</url> </organization> <licenses> <license> <name>GNU GPLv3</name> <url>https://www.gnu.org/licenses/gpl-3.0.en.html</url> <distribution>repo</distribution> </license> </licenses> <developers> <developer> <id>mygithublogin</id> <name>FirstName LastName</name> <url>https://mywebpage.net</url> <roles> <role>founder</role> <role>lead</role> <role>architect</role> <role>developer</role> <role>debugger</role> <role>tester</role> <role>maintainer</role> <role>support</role> </roles> </developer> </developers> <!-- Project properties --> <properties> <ezplug.version>3.20.0</ezplug.version> </properties> <!-- Project build configuration --> <build> </build> <!-- List of project's dependencies --> <dependencies> <!-- The core of Icy --> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>icy-kernel</artifactId> </dependency> <!-- The EzPlug library, simplifies writing UI for Icy plugins. --> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>ezplug</artifactId> <version>${ezplug.version}</version> </dependency> </dependencies> <!-- Link to third-party Maven repositories --> <repositories> <repository> <id>server-id</id> <name>Server's name</name> <url>Server's url</url> </repository> </repositories> <distributionManagement> <snapshotRepository> <id>server-id</id> <url>server's url</url> </snapshotRepository> <repository> <id>server-id</id> <url>server's url</url> </repository> </distributionManagement> </project>
- Plugin parent POM containing all commons information for Icy plugin
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <parent> <artifactId>parent-pom-base</artifactId> <groupId>org.bioimageanalysis.icy</groupId> <version>1.0.1</version> </parent> <artifactId>parent-pom-plugin</artifactId> <version>1.0.1</version> <packaging>pom</packaging> <name>Parent POM plugin</name> <description>Parent POM for all Icy plugin projects</description> <url>https://icy.bioimageanalysis.org/</url> <properties> <!-- Icy Plugins version --> <icy-kernel.version>2.1.0</icy-kernel.version> <ezplug.version>3.20.0</ezplug.version> <protocols.version>3.1.0</protocols.version> <three-d-active-meshes.version>1.3.2</three-d-active-meshes.version> <three-d-mesh-roi.version>1.4.7</three-d-mesh-roi.version> <active-contours.version>4.4.10</active-contours.version> <autocomplete.version>2.5.0</autocomplete.version> <blocks.version>5.3.0</blocks.version> <blockvars.version>0.1.2</blockvars.version> <color-picker-threshold.version>1.2.2</color-picker-threshold.version> <connected-components.version>4.8.7</connected-components.version> <convexify.version>2.0.5</convexify.version> <fill-holes-in-roi.version>1.3.1</fill-holes-in-roi.version> <filter-toolbox.version>4.3.1</filter-toolbox.version> <hk-means.version>2.9.2</hk-means.version> <icy-bioformats.version>6.3.1</icy-bioformats.version> <icy-insubstantial.version>7.3.8</icy-insubstantial.version> <icy-jython.version>2.8.0</icy-jython.version> <icy-vtk.version>6.3.0.1</icy-vtk.version> <java3d.version>1.6.0</java3d.version> <jama.version>1.0.2</jama.version> <javacl.version>1.0.6</javacl.version> <javadocparser.version>1.0.1</javadocparser.version> <label-extractor.version>1.5.1</label-extractor.version> <linear-programming.version>1.0.2</linear-programming.version> <mask-editor.version>1.2.1</mask-editor.version> <micromanager.version>1.9.0</micromanager.version> <micromanager-block.version>1.03</micromanager-block.version> <morphomaths.version>5.0.0</morphomaths.version> <nherve-matrix.version>1.1</nherve-matrix.version> <nherve-image-analysis-toolbox.version>1.2.1</nherve-image-analysis-toolbox.version> <nherve-toolbox.version>1.3.2</nherve-toolbox.version> <quickhull.version>1.0.2</quickhull.version> <rhino.version>1.7.6</rhino.version> <rigid-registration.version>1.3.1</rigid-registration.version> <roi-blocks.version>1.4.7</roi-blocks.version> <roi-inclusion-analysis.version>1.3.2</roi-inclusion-analysis.version> <roi-itensify-evolution.version>2.0.1</roi-itensify-evolution.version> <roi-pool.version>1.0.5</roi-pool.version> <roi-statistics.version>5.8.12</roi-statistics.version> <roi-tagger.version>1.0.2</roi-tagger.version> <rsyntaxtextarea.version>2.5.1</rsyntaxtextarea.version> <sequence-blocks.version>2.0.16</sequence-blocks.version> <spot-detection-utilities.version>1.1.6</spot-detection-utilities.version> <spot-detector.version>1.7.1</spot-detector.version> <spot-tracking.version>3.4.6</spot-tracking.version> <spot-tracking-blocks.version>3.4.6</spot-tracking-blocks.version> <texture-segmentation.version>1.2.1</texture-segmentation.version> <thresholder.version>3.5.1</thresholder.version> <track-manager.version>1.4.6</track-manager.version> <vecmath.version>1.6.1</vecmath.version> <workbooks.version>3.4.10</workbooks.version> </properties> <!-- POM profiles --> <profiles> <profile> <id>deploy-dev</id> <!-- Properties for this profile --> <build> <defaultGoal>clean deploy</defaultGoal> </build> <!-- Repository link for deployment --> <distributionManagement> <repository> <id>icy-dev</id> <name>Icy's Nexus</name> <url>https://icy-nexus-dev.pasteur.cloud/repository/icy-plugins/</url> </repository> <snapshotRepository> <id>icy-dev</id> <name>Icy Dev's Nexus</name> <url>https://icy-nexus-dev.pasteur.cloud/repository/icy-plugins/</url> </snapshotRepository> </distributionManagement> </profile> <profile> <id>deploy-release</id> <!-- Properties for this profile --> <build> <defaultGoal>clean deploy</defaultGoal> </build> <!-- Repository link for deployment --> <distributionManagement> <repository> <id>icy-prod</id> <name>Icy's Nexus</name> <url>https://icy-nexus.pasteur.fr/repository/icy-plugins/</url> </repository> </distributionManagement> </profile> </profiles> <!-- List of main dependencies --> <dependencyManagement> <dependencies> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>icy-kernel</artifactId> <version>${icy-kernel.version}</version> </dependency> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>ezplug</artifactId> <version>${ezplug.version}</version> </dependency> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>protocols</artifactId> <version>${protocols.version}</version> </dependency> </dependencies> </dependencyManagement> <!-- Required plugins --> <dependencies> <dependency> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>icy-kernel</artifactId> </dependency> </dependencies> <!-- Icy Maven repository (to find parent POM) --> <repositories> <repository> <id>icy</id> <name>Icy's Nexus</name> <url>https://icy-nexus.pasteur.fr/repository/Icy/</url> </repository> </repositories> <!-- Icy Maven repositories for POM deployment --> <distributionManagement> <snapshotRepository> <id>icy-dev</id> <name>Icy Dev's Nexus</name> <url>https://icy-nexus-dev.pasteur.cloud/repository/icy-core/</url> </snapshotRepository> <repository> <id>icy-prod</id> <name>Icy's Nexus</name> <url>https://icy-nexus.pasteur.fr/repository/icy-core/</url> </repository> </distributionManagement> </project>
- Base parent POM containing all commons information for any Icy project
<?xml version="1.0" encoding="UTF-8"?> <project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.bioimageanalysis.icy</groupId> <artifactId>parent-pom-base</artifactId> <version>1.0.1</version> <packaging>pom</packaging> <name>Parent POM base</name> <description>Common parent POM for all Icy related projects</description> <url>https://icy.bioimageanalysis.org/</url> <inceptionYear>2020</inceptionYear> <organization> <name>Institut Pasteur</name> <url>https://www.pasteur.fr/</url> </organization> <licenses> <license> <name>GNU GPLv3</name> <url>https://www.gnu.org/licenses/gpl-3.0.en.html</url> <distribution>repo</distribution> </license> </licenses> <developers> <developer> <id>stef</id> <name>Stéphane Dallongeville</name> <url>https://icy.bioimageanalysis.org/author/stef/</url> <roles> <role>founder</role> <role>lead</role> <role>developer</role> <role>debugger</role> <role>reviewer</role> <role>support</role> <role>maintainer</role> </roles> </developer> <developer> <id>jtinevez</id> <name>Jean-Yves Tinevez</name> <url>https://icy.bioimageanalysis.org/author/tinevez/</url> <roles> <role>lead</role> <role>reviewer</role> <role>support</role> </roles> </developer> <developer> <id>dgonzalez</id> <name>Daniel Felipe González Obando</name> <url>https://icy.bioimageanalysis.org/author/danyfel80/</url> <roles> <role>developer</role> <role>debugger</role> <role>maintainer</role> <role>support</role> </roles> </developer> <developer> <id>atournay</id> <name>Amandine Tournay</name> <roles> <role>developer</role> <role>maintainer</role> </roles> </developer> </developers> <mailingLists> <mailingList> <name>Icy</name> <post>icy-all@pasteur.fr</post> </mailingList> </mailingLists> <issueManagement> <system>GitLab</system> <url>https://gitlab.pasteur.fr/xx/issues</url> </issueManagement> <properties> <!-- Project Information --> <project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> <jdk.version>[1.8,)</jdk.version> <!-- Compile information --> <maven.compiler.source>1.8</maven.compiler.source> <maven.compiler.target>1.8</maven.compiler.target> <!-- Maven Plugins version --> <maven-compiler-plugin.version>3.2</maven-compiler-plugin.version> <maven-resources-plugin.version>3.1.0</maven-resources-plugin.version> <maven-source-plugin.version>3.2.1</maven-source-plugin.version> <maven-javadoc-plugin.version>3.2.0</maven-javadoc-plugin.version> <maven-dependency-plugin.version>3.1.1</maven-dependency-plugin.version> <maven-jar-plugin.version>3.2.0</maven-jar-plugin.version> <maven-enforcer-plugin.version>3.0.0-M3</maven-enforcer-plugin.version> <maven-help-plugin.version>3.2.0</maven-help-plugin.version> <!-- Maven plugin properties --> <maven-source-plugin.includePom>true</maven-source-plugin.includePom> <maven-jar-plugin.includeMavenDescriptor>false</maven-jar-plugin.includeMavenDescriptor> </properties> <!-- POM profiles --> <profiles> <profile> <id>compile-project</id> <activation> <activeByDefault>true</activeByDefault> </activation> <!-- Properties for this profile --> <build> <defaultGoal>clean install</defaultGoal> </build> </profile> </profiles> <build> <finalName>${project.artifactId}-${project.version}</finalName> <!-- List of pre-configured Maven plugins --> <pluginManagement> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>${maven-compiler-plugin.version}</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-resources-plugin</artifactId> <version>${maven-resources-plugin.version}</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-dependency-plugin</artifactId> <version>${maven-dependency-plugin.version}</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <version>${maven-jar-plugin.version}</version> <configuration> <outputDirectory>${outputJar}</outputDirectory> <archive> <addMavenDescriptor>${maven-jar-plugin.includeMavenDescriptor}</addMavenDescriptor> </archive> </configuration> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-source-plugin</artifactId> <version>${maven-source-plugin.version}</version> <configuration> <outputDirectory>${outputJar}</outputDirectory> <includePom>${maven-source-plugin.includePom}</includePom> </configuration> <executions> <execution> <id>attach-sources</id> <goals> <goal>jar-no-fork</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-javadoc-plugin</artifactId> <version>${maven-javadoc-plugin.version}</version> <configuration> <outputDirectory>${outputJar}</outputDirectory> </configuration> <executions> <execution> <id>attach-javadoc</id> <goals> <goal>jar</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-help-plugin</artifactId> <version>${maven-help-plugin.version}</version> <executions> <execution> <id>show-current-profile</id> <phase>compile</phase> <goals> <goal>active-profiles</goal> </goals> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-enforcer-plugin</artifactId> <version>${maven-enforcer-plugin.version}</version> <dependencies> <dependency> <groupId>org.codehaus.mojo</groupId> <artifactId>extra-enforcer-rules</artifactId> <version>1.3</version> </dependency> </dependencies> <executions> <execution> <id>enforce-rules</id> <goals> <goal>enforce</goal> </goals> </execution> </executions> <configuration> <rules> <!-- Check if there is duplicate classes --> <!--<banDuplicateClasses> <message>No Duplicate Classes Allowed! - For duplicate transitive dependencies, add dependency exclusions. - For duplications between direct dependencies, resolve or add ignored classes to this rule's configuration.</message> <ignoreClasses> <ignoreClass>org.pushingpixels.*</ignoreClass> <ignoreClass>org.jdesktop.*</ignoreClass> <ignoreClass>org.slf4j.*</ignoreClass> </ignoreClasses> <findAllDuplicates>true</findAllDuplicates> </banDuplicateClasses> --> <!-- Prevent circular dependencies --> <!--<banCircularDependencies/> --> <!-- Prevent usage of snapshots dependencies in the project --> <requireReleaseDeps> <message>Your dependency cannot be a snapshot</message> </requireReleaseDeps> </rules> <fail>true</fail> </configuration> </plugin> </plugins> </pluginManagement> <!-- Required Maven plugins --> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-enforcer-plugin</artifactId> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-source-plugin</artifactId> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-javadoc-plugin</artifactId> </plugin> </plugins> </build> <!-- Link to third-party Maven repositories --> <repositories> <repository> <id>ome-repo</id> <name>OME repository</name> <url>https://artifacts.openmicroscopy.org/artifactory/ome.releases/</url> </repository> <repository> <id>geomajas</id> <name>Geomajas</name> <url>http://maven.geomajas.org/</url> </repository> <repository> <id>icy</id> <name>Icy's Nexus</name> <url>https://icy-nexus.pasteur.fr/repository/Icy/</url> </repository> </repositories> <!-- Icy Maven repositories for POM deployment --> <distributionManagement> <snapshotRepository> <id>icy-dev</id> <name>Icy Dev's Nexus</name> <url>https://icy-nexus-dev.pasteur.cloud/repository/icy-core/</url> </snapshotRepository> <repository> <id>icy-prod</id> <name>Icy's Nexus</name> <url>https://icy-nexus.pasteur.fr/repository/icy-core/</url> </repository> </distributionManagement> </project>